1 2 3 4 5 6 7 8 9 10 11 12
| <?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent">
<ListView android:id="@+id/menu" android:layout_width="match_parent" android:layout_height="wrap_content"> </ListView>
</LinearLayout>
|
这里使用LinearLayout是为了让图片跟文本能够显示在同一排上
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| <?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent">
<LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center" > <ImageView android:id="@+id/pic" android:layout_width="wrap_content" android:layout_height="wrap_content" />
<TextView android:id="@+id/menu_listview" android:layout_width="wrap_content" android:layout_height="wrap_content" android:padding="45dp" android:textSize="40sp" android:textStyle="bold" android:singleLine="true" android:textAppearance="?android:attr/textAppearanceButton" /> </LinearLayout>
</LinearLayout>
|
3.编写展示弹窗方法
在MainActivity.java中编写方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| BottomSheetDialog dialog = null; private List<Map<String, Object>> dataList; private String[] array; private void showMenu(){ dialog = new BottomSheetDialog(MainActivity.this); View view = LayoutInflater.from(MainActivity.this) .inflate(R.layout.menu,null); ListView listView = view.findViewById(R.id.menu); dataList = new ArrayList<Map<String, Object>>(); SimpleAdapter adapter = new SimpleAdapter(MainActivity.this, getData(), R.layout.menu_listview, new String[]{"pic", "name"}, new int[]{R.id.pic, R.id.menu_listview}); listView.setAdapter(adapter); dialog.setContentView(view); dialog.setCancelable(false); dialog.show(); listView.setOnItemClickListener(new AdapterView.OnItemClickListener() { @Override public void onItemClick(AdapterView<?> adapterView, View view, int i, long l) { Toast.makeText(MainActivity.this, "你点击了" + array[i], Toast.LENGTH_SHORT).show(); dialog.dismiss(); } }); }
|
绑定单击事件还可以这样写
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| listView.setOnItemClickListener(new AdapterView.OnItemClickListener() { @Override public void onItemClick(AdapterView<?> adapterView, View view, int i, long l) { switch (i){ case 0: Toast.makeText(MainActivity.this,"你点击了"+i+"按钮",Toast.LENGTH_SHORT).show(); break; case 1: Toast.makeText(MainActivity.this,"你点击了"+i+"按钮",Toast.LENGTH_SHORT).show(); break; case 2: Toast.makeText(MainActivity.this,"你点击了"+i+"按钮",Toast.LENGTH_SHORT).show(); break; case 3: Toast.makeText(MainActivity.this,"你点击了"+i+"按钮",Toast.LENGTH_SHORT).show(); break; case 4: Toast.makeText(MainActivity.this,"你点击了"+i+"按钮",Toast.LENGTH_SHORT).show(); break; } } });
|
4.编写方法,将图片和文本绑定在一起
1 2 3 4 5 6 7 8 9 10 11 12 13
| private List<Map<String, Object>> getData() { int[] arr_pic = {R.drawable.open_door, R.drawable.light, R.drawable.cabinet, R.drawable.close}; array = new String[]{"开门", "开灯", "待添加", "关闭"}; for(int i = 0; i < array.length; i++) { Map<String, Object> map = new HashMap<String, Object>(); map.put("pic", arr_pic[i]); map.put("name", array[i]); dataList.add(map); } return dataList; }
|
5.监听弹窗dismiss事件
有时候需要在弹窗dismiss后修改值或者进行一些操作,BottomSheetDialog也给我们提供了方法
1 2 3 4 5 6
| dialog.setOnDismissListener(new DialogInterface.OnDismissListener() { @Override public void onDismiss(DialogInterface dialogInterface) { } });
|
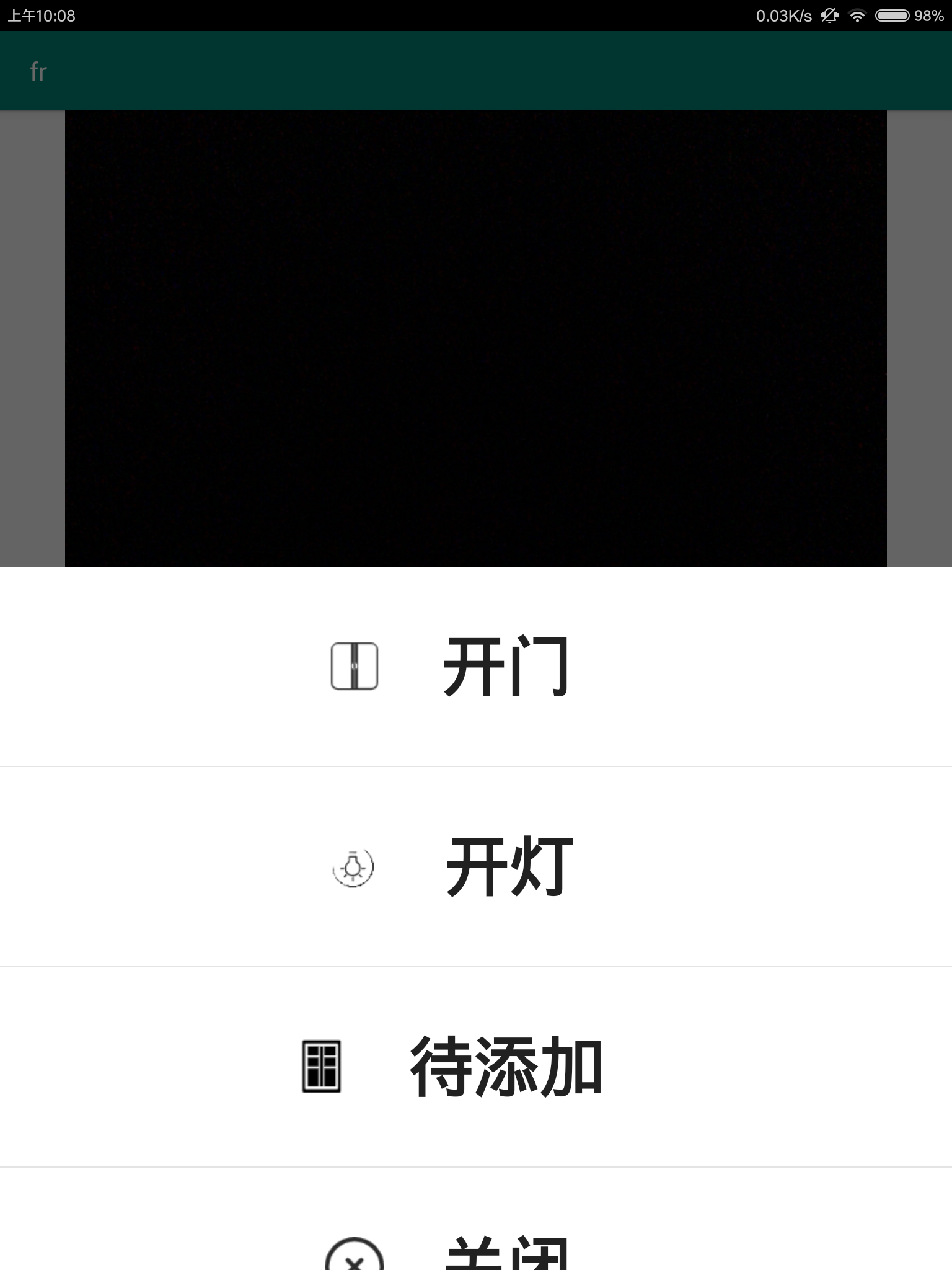