1.创建一个Gitee/Github第三方应用
这里以Gitee为例
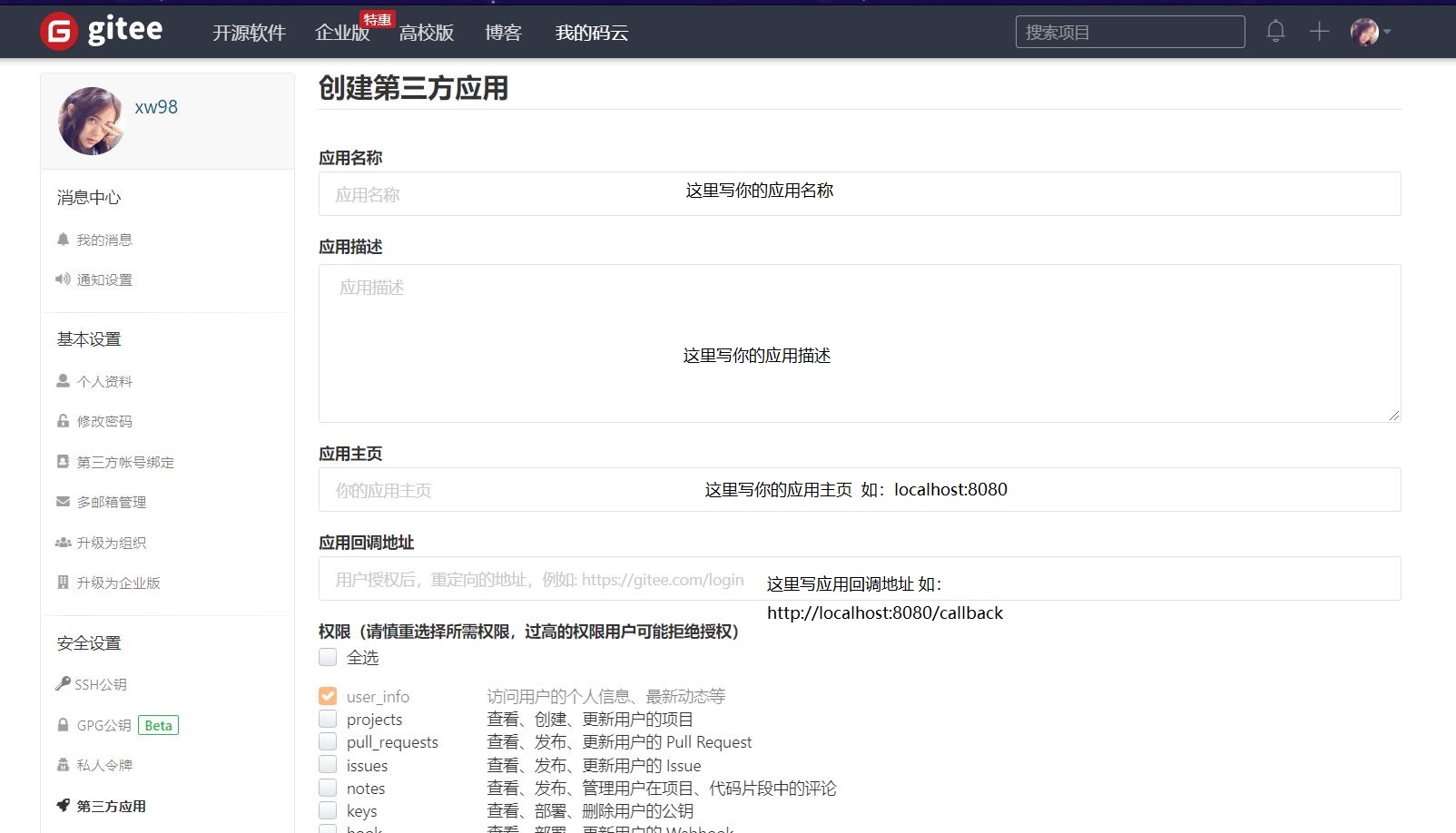
2.新建两个java类用来储存获取的Gitee/Github用户信息和获取token所需的参数
储存Github/Gitee用户信息类,生成get/set方法,我这里为了省空间就不写了
1 2 3 4 5 6
| public class GithubUser { private String name; //用户名称 private String id; //用户ID private String bio; //用户描述 private String avatar_url; //用户头像地址 }
|
储存获取token所需的参数类,生成get/set方法。
1 2 3 4 5 6 7 8
| public class AccessTokenDTO { private String client_id; //client_id private String client_secret; //client_secret private String code; //code码 private String redirect_uri; //回调地址 //private String state; //Github需要这个参数,Gitee不需要 private String grant_type; //Github不需要这个参数,Gitee需要 }
|
3.导入两个包,方便后续使用
在pom文件中导入两个包
1 2 3 4 5 6 7 8 9 10 11 12 13
| <!-- 方便发送get/post请求 --> <dependency> <groupId>com.squareup.okhttp3</groupId> <artifactId>okhttp</artifactId> <version>3.14.1</version> </dependency>
<!-- json和String类型快速转换 --> <dependency> <groupId>com.alibaba</groupId> <artifactId>fastjson</artifactId> <version>1.2.57</version> </dependency>
|
4.获取用户信息
4.1 在需要登录的位置添加链接
Github地址https://github.com/login/oauth/authorize?client_id=创建应用给的id&redirect_uri=回调地址&scope=user&state=1
Gitee地址https://gitee.com/oauth/authorize?client_id=创建应用给的id&redirect_uri=回调地址&response_type=code
4.2 新建一个控制器类
发送Git请求获取code码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42
| @Autowired private GithubProvider githubProvider;
@Autowired private AuthorizeService authorizeService;
@Value("你的clientId") private String clientId;
@Value("你的clientSecret") private String clientSecret;
@Value("你的回调地址") private String redirectUri;
@GetMapping("/callback") public String callback(@RequestParam(name = "code") String code, HttpServletResponse response){//@RequestParam(name = "state") String state, Github需要这个参数 AccessTokenDTO accessTokenDTO = new AccessTokenDTO(); accessTokenDTO.setClient_id(clientId); accessTokenDTO.setClient_secret(clientSecret); accessTokenDTO.setCode(code); accessTokenDTO.setRedirect_uri(redirectUri); accessTokenDTO.setGrant_type("authorization_code");//Gitee需要这个参数 //accessTokenDTO.setState(state); //Github需要这个参数 String accessToken = githubProvider.getAccessToken(accessTokenDTO);//获取token方法 GithubUser githubUser = githubProvider.getUser(accessToken); //获取User信息方法 if (githubUser != null && githubUser.getId() != null){ //如果不为空,插入数据库 User user = new User(); String token = UUID.randomUUID().toString(); user.setName(githubUser.getName()); user.setToken(token); user.setAccountId(githubUser.getId()); user.setAvatarUrl(githubUser.getAvatar_url()); authorizeService.insertUser(user); response.addCookie(new Cookie("token", token)); //添加cookie return "redirect:/"; //返回主页 }else { //登录失败,重新登录 return "redirect:/"; } }
|
4.3 新建一个类,写获取token的方法和获取User信息的方法
携带参数发送POST请求获取token
获取token的方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| public String getAccessToken(AccessTokenDTO accessTokenDTO){ MediaType mediaType = MediaType.get("application/json; charset=utf-8"); OkHttpClient client = new OkHttpClient();
RequestBody body = RequestBody.create(mediaType, JSON.toJSONString(accessTokenDTO)); Request request = new Request.Builder() .url("https://gitee.com/oauth/token")//https://github.com/login/oauth/access_token .post(body) .build(); try (Response response = client.newCall(request).execute()) { String string = response.body().string(); JSONObject jsonObject = JSONObject.parseObject(string); //将String转换为json串 String token = jsonObject.getString("access_token"); //获取json串中key为access_token的值 //String token = string.split("&")[0].split("=")[1];//这里Github和Gitee不同,Github返回的是一个地址,Gitee返回的是json串。 return token; } catch (Exception e) { e.printStackTrace(); } return null; }
|
使用token获取用户数据
获取User信息的方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| public GithubUser getUser(String accessToken){ OkHttpClient client = new OkHttpClient(); Request request = new Request.Builder() .url("https://gitee.com/api/v5/user?access_token=" + accessToken)//https://api.github.com/user?access_token= .build(); try { Response response = client.newCall(request).execute(); String string = response.body().string(); GithubUser githubUser = JSON.parseObject(string, GithubUser.class); return githubUser; } catch (IOException e) { } return null; }
|
以上就是调用Gitee/Github API实现第三方登录流程